Unfortunately, it doesn't run on FreeBSD, but Cadega, the Windows game platform for Linux from Transgaming, looks pretty cool if you're running a supported Linux distro. It emulates DirectX so that you can run a plethora of Windows games directly on Linux. Cool!
TransGaming.Org
Thursday, December 15, 2005
Wednesday, November 16, 2005
FreeBSD Wallpaper
A good variety of FreeBSD (and other BSDen) wallpapers, in sizes from 800x600 to 1920x1200, all in the new graphic
.
daemonisch.be - Wallpaper f�eBSD, NetBSD & OpenBSD
.
daemonisch.be - Wallpaper f�eBSD, NetBSD & OpenBSD
Tuesday, November 15, 2005
Sharing Linux swap space
I've linked about this before, but here's another page that shows you, in excruciating detail, how to have Linux & FreeBSD share a swap partition.
The Linux+FreeBSD mini-HOWTO
The Linux+FreeBSD mini-HOWTO
Friday, November 11, 2005
FreeBSD 6.0!
Wow, I never even got a 5.x release to work right! And here is 6.0 released - blink and you miss a major version, I guess. I'll give this release a try too. Maybe it'll work with my KVM switch - ha!
FreeBSD 6.0-RELEASE Announcement
FreeBSD 6.0-RELEASE Announcement
PC-BSD 1.0, rc1
The PC-BSD releases are coming hot and heavy now. I actually had a great deal of luck with the previous version. It installed and ran just like it was supposed to. I'll start from scratch with this one and see how it does. Still wish it would work with my KVM switch, though.
PC-BSD 1.0rc1
PC-BSD 1.0rc1
Tuesday, November 1, 2005
New logo for FreeBSD
FreeBSD has a new logo:

It's pretty. Mind you, they've gone to great lengths to assure everyone that they have not dropped their mascot:
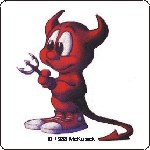
FAQ: What is the little guy's name?
History of the BSD Daemon
FreeBSD logo design competition : result

It's pretty. Mind you, they've gone to great lengths to assure everyone that they have not dropped their mascot:
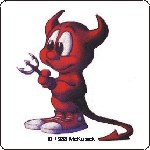
FAQ: What is the little guy's name?
History of the BSD Daemon
FreeBSD logo design competition : result
Friday, October 28, 2005
A Programmer's Cautionary Tale
Warning - geek jargon ahead, including light C code reading and other programming nerdliness.
I've been writing code since 1979 (doh!) and writing C (and, later, C++) code since the early 80s. I've seen and written, nearly every possible way you can write bad code. But I'm pretty good about learning from my mistakes, and I like to think I don't make the same mistake twice, once the errors of my ways are pointed out.
But some programming mistakes are just hard to avoid. And that's why we have compilers as our first line of defense. There's lots and lots of code that they just won't allow through, and lots of code they will at least complain about.
Yes, you can use other tools to check your source code. The most common tool that reads C and C++ source code to warn you about dubious constructs is called lint. The problem is, it is a beast to use and a bear to configure, especially if you are trying to run it on already written code. As it is supposed to, lint complains about everything, no matter how trivial a problem it may or may not be. So you spend a lot of time getting its settings so that it reflects how aggressive you want it to be, and you keep doing this. This means it generally isn't used, often to the detriment of better code.
There are many other tools that do lint-like things, and even more. I've used Parasoft's tools and have found them to be very nice. While we were at a local Linux show, we talked to a company that sold a very comprehensive source code analsysis tool.
Each of these commercial tools has a big drawback - cost. At US$1000 and up, they are not minor investments. Now, admittedly, US$1000 is not that much to a company. I get paid a pretty penny to code, as do my co-workers and a US$1000 tool that saved 10 hours of work would begin paying for itself. But it still is a hard thing to justify, as Jack Ganssle talks about. And each takes time to begin using, and to continue to use in order to be most effective.
So the front line of code analysis remains the compiler. It is, after all, the final arbiter of what your code can look like. So we've come to expect some small level of meta-analysis, warning us of dubious code, like unpredicitable side effects, unused variables, using an assignment in an if statement, etc. And, unfortunately, I got bit by a couple of GCC's pecadillos.
GCC is the standard compiler in the Linux, *BSD and Unix world. Freely available, incredibly powerful, it is really just a front end to a whole suite of compilation and linking tools. It has dozens and dozens of options, but the one we're concerned about here is the -Wall, which turns on all warnings, so it will be persnickety about your code. So I gave it some code that looked something like this:
Everything is fine, and going according to plan. A little later, it turned out that CheckSOmething should be tried a few times before it is assumed there is a problem. So I quickly (ahh, the key word) changed it to be:
Do you see the problem? At first glance, it looked okay, so off it went. No complaints from GCC, the code worked perfectly and everyone was happy.
Then, months later, I got a complaint that the code wasn't working any more. It wasn't recovering fromthe error correctly. A quick re-scan of the code and sure enough, the problem is pretty evident. But my question then became, why didn't GCC complain about the error? It's a pretty common error, and one that compilers have been warning about for decades. Turns out there are two intertwined reasons that this bug remained hidden under a rock for so long, only rearing its ugly head when the error case cropped up.
So I got nailed by probably the oldest programming bugaboo in the book - using an "uninitialized" variable. It worked in the normal case, because I was using the -g flag, which set rv to be 0, which, you'll remember is the OK status. So the loop never got executed, CheckSomething is never called, and things continued along their merry way, which is okay unless there is a problem and you want to try to fix it. You'll never know about it.
So the obvious fix is:
And now we're golden. We're going to call CheckSomething at least once, and now we'll correctly get the return value for checking later. So the moral of the story is to use -Wall but to be sure to compile the final version with -O to get all the warnings. And to look into getting a C++ code analyzer!
I've been writing code since 1979 (doh!) and writing C (and, later, C++) code since the early 80s. I've seen and written, nearly every possible way you can write bad code. But I'm pretty good about learning from my mistakes, and I like to think I don't make the same mistake twice, once the errors of my ways are pointed out.
But some programming mistakes are just hard to avoid. And that's why we have compilers as our first line of defense. There's lots and lots of code that they just won't allow through, and lots of code they will at least complain about.
Yes, you can use other tools to check your source code. The most common tool that reads C and C++ source code to warn you about dubious constructs is called lint. The problem is, it is a beast to use and a bear to configure, especially if you are trying to run it on already written code. As it is supposed to, lint complains about everything, no matter how trivial a problem it may or may not be. So you spend a lot of time getting its settings so that it reflects how aggressive you want it to be, and you keep doing this. This means it generally isn't used, often to the detriment of better code.
There are many other tools that do lint-like things, and even more. I've used Parasoft's tools and have found them to be very nice. While we were at a local Linux show, we talked to a company that sold a very comprehensive source code analsysis tool.
Each of these commercial tools has a big drawback - cost. At US$1000 and up, they are not minor investments. Now, admittedly, US$1000 is not that much to a company. I get paid a pretty penny to code, as do my co-workers and a US$1000 tool that saved 10 hours of work would begin paying for itself. But it still is a hard thing to justify, as Jack Ganssle talks about. And each takes time to begin using, and to continue to use in order to be most effective.
So the front line of code analysis remains the compiler. It is, after all, the final arbiter of what your code can look like. So we've come to expect some small level of meta-analysis, warning us of dubious code, like unpredicitable side effects, unused variables, using an assignment in an if statement, etc. And, unfortunately, I got bit by a couple of GCC's pecadillos.
GCC is the standard compiler in the Linux, *BSD and Unix world. Freely available, incredibly powerful, it is really just a front end to a whole suite of compilation and linking tools. It has dozens and dozens of options, but the one we're concerned about here is the -Wall, which turns on all warnings, so it will be persnickety about your code. So I gave it some code that looked something like this:
#include <stdlib.h>
const int OK=0;
const int ERROR=1;
const int MAX_RETRIES=3;
int main( int /*argc*/, char* /*argv[]*/ )
{
int rv = CheckSomething();
if ( rv == ERROR )
{ // error return, try to work around it
// ... do some recovery stuff, give up if it doesn't work
}
// ... everything's okay, so carry on
return 0;
}
Everything is fine, and going according to plan. A little later, it turned out that CheckSOmething should be tried a few times before it is assumed there is a problem. So I quickly (ahh, the key word) changed it to be:
#include <stdlib.h>
const int OK=0;
const int ERROR=1;
const int MAX_RETRIES=3;
int main( int /*argc*/, char* /*argv[]*/ )
{
int rv;
for ( int rcnt=0; rcnt < MAX_RETRIES && rv != OK; ++rcnt )
rv = ();
if ( rv == ERROR )
{ // error return, try to work around it
// ... do some recovery stuff, give up if it doesn't work
}
// ... everything's okay, so carry on
return 0;
}
Do you see the problem? At first glance, it looked okay, so off it went. No complaints from GCC, the code worked perfectly and everyone was happy.
Then, months later, I got a complaint that the code wasn't working any more. It wasn't recovering fromthe error correctly. A quick re-scan of the code and sure enough, the problem is pretty evident. But my question then became, why didn't GCC complain about the error? It's a pretty common error, and one that compilers have been warning about for decades. Turns out there are two intertwined reasons that this bug remained hidden under a rock for so long, only rearing its ugly head when the error case cropped up.
- GCC zero initializes automatic variables when built in 'debug' mode, by using the -g flag. Normally, automatic variables (ie., ones that are declared inside the program and are not globals) are not initialized to any predictable value by the compiler; the programmer needs to do it specifically. But GCC sets them to zero, but only when built for debugging, not when you use the optimizer (the -O flag).
- The -Wall flag doesn't warn about using unitialized variables unless the -O flag is used. The man page says:
These warnings are possible only in optimizing compilation, because they require data flow information that is computed only when optimizing. If you don't specify -O, you simply won't get these warnings.
So I got nailed by probably the oldest programming bugaboo in the book - using an "uninitialized" variable. It worked in the normal case, because I was using the -g flag, which set rv to be 0, which, you'll remember is the OK status. So the loop never got executed, CheckSomething is never called, and things continued along their merry way, which is okay unless there is a problem and you want to try to fix it. You'll never know about it.
So the obvious fix is:
#include <stdlib.h>
const int OK=0;
const int ERROR=1;
const int MAX_RETRIES=3;
int main( int /*argc*/, char* /*argv[]*/ )
{
int rv=ERROR;
for ( int rcnt=0; rcnt < MAX_RETRIES && rv != OK; ++rcnt )
rv = CheckSomething();
if ( rv == ERROR )
{ // error return, try to work around it
// ... do some recovery stuff, give up if it doesn't work
}
// ... everything's okay, so carry on
return 0;
}
And now we're golden. We're going to call CheckSomething at least once, and now we'll correctly get the return value for checking later. So the moral of the story is to use -Wall but to be sure to compile the final version with -O to get all the warnings. And to look into getting a C++ code analyzer!
Thursday, October 27, 2005
fortune and fame
The fortune command spills out a small witticism or other interesting quote. I've talked about it before here, where I pointed out there was a FreeBSD tips fortune file.
Well, it's pretty easy to add your own quote file, but I can never remember how to do it, so I'm documenting it here.
fortune generally reads its data files from /usr/share/games/fortune - all the files that have a '-o' appended are "offensive" ones. You can pass a quote file (soon I'll tell you how to make one) on its command line and it will use that one. The special file name "all" will use all the ones in the "standard" places. Other useful options are -a, to include "offensive" quotes, -e, to consider them all to have equal sizes (otherwise it chooses which file based upon the size of the file), and the -l/-s flags to show only long or short ones.
To build your own fortune file, create a simple text file that has no extension and is a list of strings, separated by a line that has just a '%' on it. It would look something like this:
Then run the strfile command on it, which generates a .dat file with indices into this quote file for fortune to use:
So now you can run fortune on this file:
The easiest way to integrate this into the normal fortune lookup is to put it into the /usr/share/fortune directory, but that isn't usually writable by normal folks. This means you need to create an alias to do it:
The -a says to include the "offensive" ones, the -e says to chose equally among the files, the 'all' says to include all the standard ones and then I add my own file at the end.
Again, don't forget about the freebsd-tips fortune file:
gives you a fortune from the tip file. I used the backslash to avoid the fortune command getting replaced by my alias (all of this discussion assumes you are using bash for a shell). To see if it is working correctly add in the -f flag, which displays the files it will use:
Again, note the backslash to "quote" the f, so that alias expansion doesn't happen. It's a nice little shortcut if you think an alias is getting in the way.
For some reason, the FreeBSD.org man pages on the web, found at FreeBSD Hypertext Man Pages don't have the 'fortune' - probably a bug.
strfile
Well, it's pretty easy to add your own quote file, but I can never remember how to do it, so I'm documenting it here.
fortune generally reads its data files from /usr/share/games/fortune - all the files that have a '-o' appended are "offensive" ones. You can pass a quote file (soon I'll tell you how to make one) on its command line and it will use that one. The special file name "all" will use all the ones in the "standard" places. Other useful options are -a, to include "offensive" quotes, -e, to consider them all to have equal sizes (otherwise it chooses which file based upon the size of the file), and the -l/-s flags to show only long or short ones.
To build your own fortune file, create a simple text file that has no extension and is a list of strings, separated by a line that has just a '%' on it. It would look something like this:
It's amazing how many people in this world are born at third base,
and think they've hit a triple.
Albert L. Lilly III
%
Eagles may soar, but weasels don't get sucked into jet engines.
Todd C. Somers
%
There is no psychiatrist in the world like a puppy licking your face.
Ben Williams
%
Then run the strfile command on it, which generates a .dat file with indices into this quote file for fortune to use:
$ strfile quotes
So now you can run fortune on this file:
$ fortune quotes
The easiest way to integrate this into the normal fortune lookup is to put it into the /usr/share/fortune directory, but that isn't usually writable by normal folks. This means you need to create an alias to do it:
$ alias fortune="fortune -ae all ~/quotes"
The -a says to include the "offensive" ones, the -e says to chose equally among the files, the 'all' says to include all the standard ones and then I add my own file at the end.
Again, don't forget about the freebsd-tips fortune file:
$ \fortune freebsd-tips
gives you a fortune from the tip file. I used the backslash to avoid the fortune command getting replaced by my alias (all of this discussion assumes you are using bash for a shell). To see if it is working correctly add in the -f flag, which displays the files it will use:
$ \fortune -fae all ~/quotes
___% /usr/share/games/fortune
___% fortunes
___% fortunes2
___% freebsd-tips
___% murphy
___% startrek
___% zippy
___% fortunes2-o
___% limerick
___% murphy-o
___% fortunes-o
Again, note the backslash to "quote" the f, so that alias expansion doesn't happen. It's a nice little shortcut if you think an alias is getting in the way.
For some reason, the FreeBSD.org man pages on the web, found at FreeBSD Hypertext Man Pages don't have the 'fortune' - probably a bug.
strfile
Tuesday, October 25, 2005
New PC-BSD release
PC-BSD 0.8.3 has been released to the wild. Once again, I'm going to download it and give it a try on my test machine. I won't even make it work with my KVM box either. I have have a USB mouse hooked directly up to it.
PC-BSD - Personal Computing, served up BSD Style!
PC-BSD - Personal Computing, served up BSD Style!
Tuesday, October 18, 2005
portaudit
Cool port that keeps a database of all the ports that have security problems, and it won't let you install them without a manual override. I'm going to look a little more into this one!
FreshPorts -- security/portaudit
FreshPorts -- security/portaudit
Wednesday, October 12, 2005
grep help
I finally broke down and figured out how to better use the recursive grep function. Although I've been using the bash shell via Cygwin on Windows for a few months now, I'm still partial to 4NT as a command shell. There's a few nice features I wish that bash had:
I knew about grep's -R flag, but found it hard to use and couldn't figure out how to recursively search just .h files, for instance. I finally broke down and read the man page, and here's what I found out.
To recursively search for something, you can use the -R flag to grep:
To recursively check just .h files:
Where:
fgrep - "fast" grep. You can use this if you don't use any regexps in the search pattern
-R - recursively search folders, starting with this one (.)
--include="*.h" - just include *.h files. You need to put the quotes around the wildcard, or the shell will try to expand it and it won't work like you think. That's why you can't just do:
- a better directory recall
- The '...' (and beyond) facility for changing directories upwards an arbitrary number of parents
- a directory database
- A much easier to use "FOR" facility from the command line
- The FFIND /S command, which recursively searches for text in files
I knew about grep's -R flag, but found it hard to use and couldn't figure out how to recursively search just .h files, for instance. I finally broke down and read the man page, and here's what I found out.
To recursively search for something, you can use the -R flag to grep:
$ fgrep -R BIGENDIAN .
To recursively check just .h files:
$ fgrep -R --include="*.h" BIGENDIAN .
Where:
fgrep - "fast" grep. You can use this if you don't use any regexps in the search pattern
-R - recursively search folders, starting with this one (.)
--include="*.h" - just include *.h files. You need to put the quotes around the wildcard, or the shell will try to expand it and it won't work like you think. That's why you can't just do:
$ fgrep -R BIGENDIAN *.h
It will still just search local .h files, because the shell expands the wildcard before fgrep gets it. So if there are two .h files in the current directory, the command looks like this after the shell gets done with it:
$ fgrep -R BIGENDIAN foo.h bar.h
You can add multiple --includes, and there's even a --exclude you can add to exclude files of that pattern from the search.
grep man page
Tuesday, October 11, 2005
/dev/random
Looking into some random number generator stuff and I came across this page on getting /dev/random ready for generating some good random numbers. Mind you, this is just for 4.x (like my server), as 5.x and beyond have a bultin setup for doing that random number thing (see the note at the bottom of this page).
Prepping /dev/random in FreeBSD 4.x
Prepping /dev/random in FreeBSD 4.x
Thursday, October 6, 2005
New Freebsd.org home page
Whoa! I just went to the FreeBSD.org home page and found the all-new layout. It's got some nice things about it, but I surely miss the easy links to the Ports page especially. I had to go on a bit of a quest in fact to find it. FYI, you can find it under the "Get FreeBSD" button at the top, then the last item on the menu at the left.
Still, it's a pretty clean looking layout, and maybe my original shock will give way to a grudging acceptance.
The FreeBSD Project
Still, it's a pretty clean looking layout, and maybe my original shock will give way to a grudging acceptance.
The FreeBSD Project
Drupal
Yet another "Content Management Platform". I've talked about them before (like here), and here is another one, called "Drupal". Something I'd surely love to play with in my non-existent free time.
drupal.org | Community plumbing
Port description for www/drupal
drupal.org | Community plumbing
Port description for www/drupal
Monday, September 26, 2005
*BSD vs. Linux
Here's some links to some (pro-FreeBSD) pages that compare BSD to Linux. I often wonder the same myself - why did I go to BSD instead of Linux? I use Linux at work, and have installed many different Linux distros, yet I picked FreeBSD for my server. I think I like the fact that source code is still very important to the BSD community. You can always download and install something via the source code, and it is usually more painless than just about any Linux distro I've tried. I also like the model of a single source for the distribution, rather than dozens of competing development trees, although with the multiple flavors of FreeBSD these days, that might not be quite as clearcut as before.
bsd-linux-comparison
FreeBSD: An Open Source Alternative to Linux
BSD For Linux Users :: Intro
bsd-linux-comparison
FreeBSD: An Open Source Alternative to Linux
BSD For Linux Users :: Intro
GTK Theme selector
I'm just beginning to get into FreeBSD with a GUI. My server is all text mode. So I'm not really exactly sure *what* a "GTK-Theme" is, but here is a recommended little app to help you select one that you've installed:
rico_GTK-Theme-Selector Homepage
rico_GTK-Theme-Selector Homepage
Fancy GTK+ apps
There's a PC-BSD forum post that describes how to get "fancier" looking GTK+ (a GUI toolkit used by KDE) apps
Of course, if you're like me, you'll probably want to make it from the source:
According to the FreeBSD ports page:
Fancy nicer looking GTK+ apps? Apps that fall into this category include Firefox and Thunderbird. Simply run the following command from a console (as root) and restart your x session:
# pkg_add -r gtk-qt-engine
Of course, if you're like me, you'll probably want to make it from the source:
# cd /usr/ports/x11-themes/gtk-qt-engine
# make && make install && make clean
According to the FreeBSD ports page:
GTK-QT Theme Engine allows GTK2 apps to use QT (KDE) themes
More PC-BSD
Here's yet another release of PC-BSD, this time 0.8.2. It fixes a few more installation problems, including the one I'm seeing, where the"mountroot" command doesn't work when rebooting after installation. It says that the online update will pick up the changes but either that isn't working, or my installation is the 0.8 installation. It's too bad they don't label these things somewhere. You'd think a README on the root level of the ISO would say exactly which version of the ISO you have?
Anyway, a little update on my BSD follies. I still have my KVM mouse problems (sigh), but I've gone and plugged in another mouse to this box in order to get around them. My PC-BSD install went pretty smoothly, but then I ran into the above mentioned 'mountroot' problem. It seems to be trying to get the OS from the wrong slice (partition). Luckily, I barely remembered both where I installed it and the nomenclature for specifying it. I think they are having a problem with it defaulting to one (ad1s1a) no matter where you actually installed it (in my case, ad6s1a). It's 'ad6' because this is such an old box, it has a funky "fast" controller, back in the days when a UDMA 66 was fast, that isn't part of the normal 4 IDE controllers.
Anyway, I get the following error when I boot PC-BSD:
Then it gives me a little info on how to use the manual input portion of mountroot (whatever that is - it isn't documented in the handbook as far as I can tell). By using the '?' command, I get a list of the possible boot devices, and there I can see 'ad6s1a', which is the 'a' (root file system) partition (in BSD speak) of the first slice (s1) of the 7th IDE device (like I said, it gets put way past the normal 0-3 due to the funky controller). So, if I type in:
I'm off and running.
But I'm also downloading this 0.8.2 version. I will start again from scratch. I have a big hard drive (120gb) empty, so I can play there. I will probably continue to try out PC-BSD, so as to keep my hand in the BSD game, despite the wickedly annoying KVM problem.
Development Release: PC-BSD 0.8.2 (Beta)
Anyway, a little update on my BSD follies. I still have my KVM mouse problems (sigh), but I've gone and plugged in another mouse to this box in order to get around them. My PC-BSD install went pretty smoothly, but then I ran into the above mentioned 'mountroot' problem. It seems to be trying to get the OS from the wrong slice (partition). Luckily, I barely remembered both where I installed it and the nomenclature for specifying it. I think they are having a problem with it defaulting to one (ad1s1a) no matter where you actually installed it (in my case, ad6s1a). It's 'ad6' because this is such an old box, it has a funky "fast" controller, back in the days when a UDMA 66 was fast, that isn't part of the normal 4 IDE controllers.
Anyway, I get the following error when I boot PC-BSD:
Mounting root from ufs:/dev/ad1s1a
setrootbyname failed
ffs_mountroot: can't find rootvp
Root mount failed: 6
Then it gives me a little info on how to use the manual input portion of mountroot (whatever that is - it isn't documented in the handbook as far as I can tell). By using the '?' command, I get a list of the possible boot devices, and there I can see 'ad6s1a', which is the 'a' (root file system) partition (in BSD speak) of the first slice (s1) of the 7th IDE device (like I said, it gets put way past the normal 0-3 due to the funky controller). So, if I type in:
mountroot> ufs:ad6s1a
I'm off and running.
But I'm also downloading this 0.8.2 version. I will start again from scratch. I have a big hard drive (120gb) empty, so I can play there. I will probably continue to try out PC-BSD, so as to keep my hand in the BSD game, despite the wickedly annoying KVM problem.
Development Release: PC-BSD 0.8.2 (Beta)
Thursday, September 22, 2005
Blob meme - report on an old post
Via Pharyngula
Rules:
Rules:
- Go into your archive.
- Find your 23rd post (or closest to).
That would be from June 2003, FreeBSD Splash Screens - Find the fifth sentence (or closest to).
- Post the text of the sentence in your blog along with these instructions.
This page has lots more splash and desktop screens, including some vaguely erotic and futuristic BSD Daemons:
Tuesday, September 20, 2005
DesktopBSD - Yet Another BSD Distro
Well, I figured I would try and install a full, GUI version of FreeBSD on my tertiary computer. It's an oldie but goodie - 800mhz overclocked Celeron, 256mb RAM, but a big hard drive. The idea, as I believe I've mentioned before, is to run a backup server, something that runs a Bacula server to back up all the other machines on my network. And to have a local FreeBSD machine to hack around on, as my FreeBSD server is now off living its own life in NH these days.
I still haven't had much luck with my KVM switch. PC-BSD just doesn't seem to recognize the mouse, and DragonFly BSD seems to rudimentary to play with. It's a long-standing problem with FreeBSD for some reason - it just doesn't see a mouse when it is hooked up via a KVM switch. Looking back through my notes here, I see where I once got FreeSBIE to work by jiggling when X start up, but I can't seem to even get that to work any more :-(
Anyway, here's Yet Another BSD distro, called DesktopBSD. I'll give it a shot...
DesktopBSD: Home
I still haven't had much luck with my KVM switch. PC-BSD just doesn't seem to recognize the mouse, and DragonFly BSD seems to rudimentary to play with. It's a long-standing problem with FreeBSD for some reason - it just doesn't see a mouse when it is hooked up via a KVM switch. Looking back through my notes here, I see where I once got FreeSBIE to work by jiggling when X start up, but I can't seem to even get that to work any more :-(
Anyway, here's Yet Another BSD distro, called DesktopBSD. I'll give it a shot...
DesktopBSD: Home
Monday, September 19, 2005
New PC-BSD release
My current favorite for user-frienlyd FreeBSD installations is PC-BSD. It actually works on my my truly lagging edge computer, which is a miracle in itself, especially the problems that my KVM (Keyboard/Video/Mouse) switch gives the BSD X Windows. Still not solved, as far as I'm concerned, but this distro has actually worked in the past. I'm going to put some serious effort into getting it up and running on my 800mhz(!) Celeron and see if I can't make it the backup server I was planning on.
Anyway, they just came out with version 0.8 of PC-BSD, so that's the one I'm going to go with. I downloaded the ISO and I'm ready to burn!
PC-BSD - Personal Computing, served up BSD Style!
Updated: : As a quick followup, there is now a 0.8.1 release.
Anyway, they just came out with version 0.8 of PC-BSD, so that's the one I'm going to go with. I downloaded the ISO and I'm ready to burn!
PC-BSD - Personal Computing, served up BSD Style!
Updated: : As a quick followup, there is now a 0.8.1 release.
Friday, September 16, 2005
FreeBSD Boot process
A couple of pointers to man pages that will help you follow the FreeBSD boot process. For the best explanation of all the stuff that appears when you boot FreeBSD, I will refer you to Chapter 29 "Starting and stopping the system" of The Complete FreeBSD, 4th edtion. It has a great explanation of what the system does during boot, how it goes through the process, what it is telling you and how to configure it (note: I helped proofread it and got a free copy of the book, but trust me, it's worth paying for!).
This is a description of the boot process in the man pages. Start here, read it and check out the "See Also" links: boot
Here's how to tell the boot loader the options to use and even the kernal to load the next time you restart the system: nextboot
The final stage of the "bootstrap", is the loader(8)
If you use the builtin boot manager, you can use this program to configure it: boot0cfg(8)
This is a description of the boot process in the man pages. Start here, read it and check out the "See Also" links: boot
Here's how to tell the boot loader the options to use and even the kernal to load the next time you restart the system: nextboot
The final stage of the "bootstrap", is the loader(8)
If you use the builtin boot manager, you can use this program to configure it: boot0cfg(8)
Thursday, September 15, 2005
Server Monitoring
Now that I run my main server remotely, I should probably look into monitoring software a little more. You know, software that can tell you at a glance if things are working or not. Luckily, the ISP that hosts my box, MV Communications, runs a pinger that checks to make sure the box is up and responding, so that's a basic check that covers 90% of the problems.
Anyway, I guess I should search my own archives, as I already have a post that describes two of them (Nagios and Big Brother):
Monitoring Programs
There are two others I should look into:
Anyway, I guess I should search my own archives, as I already have a post that describes two of them (Nagios and Big Brother):
Monitoring Programs
There are two others I should look into:
Wednesday, September 14, 2005
FreeBSD Snapshots
Gee, a lot of cool links in today's reading of the FreeBSD-questions list! I'm way behind (only up to early August!), but I still get lots and lots of cool info and links. This time, it is a discussion on a separate partition for /boot (not really possible, I gather) and snapshots. Here's a page talking about soft updates and snapshots. They sound pretty cool, although only applicable to 5.x, so I'll need to get my new version installed and running before I can play with it.
Soft Updates and Snapshots
Soft Updates and Snapshots
Postfix help
To be honest, I don't use postfix, still in the sendmail days. And even funnier, I don't even know what "TLS" and "SASL" are!-) But it seems like a very useful and complete page for setting up Postfix with "TLS and SASL", which could be a help to someone. So here goes:
Postfix with TLS and SASL
Postfix with TLS and SASL
Automounting
Here's a good long explanation of "auto-mounting", the process whereby certain volumes are automatically mounted when you try to access them, and unmounts them when they seem to be unused. You shouldn't put network drives in your fstab(5), as your boot times will be much slower and it might even hang if there are network problems. By using amd, you can mount them when you actually try to access the drive.
One thing I'd be wary of is that this is a file that is 3 years old at this point. I haven't used amd all that much, and I haven't read this file over carefully, but you need to make sure the information isn't obsolete. I've added links to the handbook and man pages for the appropriate tools, so you might want to start there and only use this file if you have additional questions.
Fun With Automounting on FreeBSD
Here are the appropriate FreeBSD Handbook (the first place to go when you have questions) and man page entries:
Network File System (NFS) - 24.3.4 Automatic Mounts with amd
amd(8)
amq
amd.conf(5)
One thing I'd be wary of is that this is a file that is 3 years old at this point. I haven't used amd all that much, and I haven't read this file over carefully, but you need to make sure the information isn't obsolete. I've added links to the handbook and man pages for the appropriate tools, so you might want to start there and only use this file if you have additional questions.
Fun With Automounting on FreeBSD
Here are the appropriate FreeBSD Handbook (the first place to go when you have questions) and man page entries:
Network File System (NFS) - 24.3.4 Automatic Mounts with amd
amd(8)
amq
amd.conf(5)
Monday, September 12, 2005
Ultimate Boot CD
A really cool diagnostic tool - the Ultimate Boot CD. It is a bootable CD-ROM that contains all kinds of diagnostic tools, from benchmarking to boot managers. Be sure to check out the Full version, which also includes the INSERT environment, which is a Knoppix derived Linux shell.
Ultimate Boot CD - Overview
Ultimate Boot CD - Overview
Thursday, August 25, 2005
Seeing all processes
'ps' is, as I'm sure you all know, a very useful command. 'ps' stands for 'process status' and it gives you information on running processes, and has almost as many options as ls. My standard ps bash alias is :
This shows all (-a) processes, swapped (-f, but only as root) processes, lots of information (-u), include "headless" apps, like daemons and the like (-x), and do it as wide as you can get (-w says 132 columns, -ww says use it as wide as you need).
Turns out, there are a couple of sysctl options that make ps more secure by not allowing "normal" users to show everything that is running. One of them is mentioned in the man page, security.bsd.see_other_uids (see the entry for the -a option). Kevin on the freebsd questions mailing list gives a cool little demo for setting and unsetting it from the commandline:
But that's a 5.x option. The 4.x option is kern.ps_showallprocs - pretty straight forward name there. And to set this so that it gets correctly set every boot time, you put it in the /etc/sysctl.conf file:
ps (5.x)
ps (4.x)
alias ps='ps -afuxww'
This shows all (-a) processes, swapped (-f, but only as root) processes, lots of information (-u), include "headless" apps, like daemons and the like (-x), and do it as wide as you can get (-w says 132 columns, -ww says use it as wide as you need).
Turns out, there are a couple of sysctl options that make ps more secure by not allowing "normal" users to show everything that is running. One of them is mentioned in the man page, security.bsd.see_other_uids (see the entry for the -a option). Kevin on the freebsd questions mailing list gives a cool little demo for setting and unsetting it from the commandline:
# sysctl -a | grep other_uid
security.bsd.see_other_uids: 1
# sudo sysctl security.bsd.see_other_uids=0
security.bsd.see_other_uids: 1 -> 0
# sysctl -a | grep other_uid
security.bsd.see_other_uids: 0
# sudo sysctl security.bsd.see_other_uids=1
security.bsd.see_other_uids: 0 -> 1
# sysctl -a | grep other_uid
security.bsd.see_other_uids: 1
But that's a 5.x option. The 4.x option is kern.ps_showallprocs - pretty straight forward name there. And to set this so that it gets correctly set every boot time, you put it in the /etc/sysctl.conf file:
kern.ps_showallprocs=1
ps (5.x)
ps (4.x)
Wednesday, August 24, 2005
Yet Another Boot Manager
There are a million boot managers out there. A boot manager, in case you didn't know, is something the OS geeks like myself use to select which OS to boot when the computer is restarted. It can be very useful for testing purposes. Say you want to see if your software works on Win98, or on some flavor of Linux. If you have the hard drive space, you create a new partition and install a completely standalong OS.
This is different than an emulator like Virtual PC or, even better, VMWare. Emulators run like just another program, and can bring the buffest hardware to its knees.
By using a boot manager, you can get a truly pristine setup. FreeBSD has its own boot manager - most non-Windows OSes will install their own, allowing you to choose between the dark side and the side of light and goodness. But the native ones are generally pretty basic, and don't give you things like partition managers, wizards, graphical interfaces, etc.
I've used a couple in my time. I used to be a huge fan of System Commander. I used it from version 1 up until last year, and was very happy with it. Easy to use, very smart, a great product.
But last year I started running into plenty of problems with it. The display would be garbled, and it just wouldn't work. I got less than satisfactory help from the VCom folks, so I started looking around.
Now I use BootIt NG from Terrabyte Unlimited. A shareware product that has worked very well for me. It's a little less easy to use, but has proven to be very powerful. While you're at the site, be sure to check out other, Windows, freeware utilities like BurnCDCC, which is a very easy to use ISO burner.
There are some freeware ones out there too. Grub is a standard one in the Linux world.
Here is GAG, a freeware one. I'll give it a shot on my test machine and see how it does:
The home page of GAG - main
This is different than an emulator like Virtual PC or, even better, VMWare. Emulators run like just another program, and can bring the buffest hardware to its knees.
By using a boot manager, you can get a truly pristine setup. FreeBSD has its own boot manager - most non-Windows OSes will install their own, allowing you to choose between the dark side and the side of light and goodness. But the native ones are generally pretty basic, and don't give you things like partition managers, wizards, graphical interfaces, etc.
I've used a couple in my time. I used to be a huge fan of System Commander. I used it from version 1 up until last year, and was very happy with it. Easy to use, very smart, a great product.
But last year I started running into plenty of problems with it. The display would be garbled, and it just wouldn't work. I got less than satisfactory help from the VCom folks, so I started looking around.
Now I use BootIt NG from Terrabyte Unlimited. A shareware product that has worked very well for me. It's a little less easy to use, but has proven to be very powerful. While you're at the site, be sure to check out other, Windows, freeware utilities like BurnCDCC, which is a very easy to use ISO burner.
There are some freeware ones out there too. Grub is a standard one in the Linux world.
Here is GAG, a freeware one. I'll give it a shot on my test machine and see how it does:
The home page of GAG - main
Emacs delete key
Something that I never quite got working right was the key mapping on my FreeBSD box, at least when I was working locally and using Emacs. The normal key mapping has the Backspace key generating a Ctrl-h, which translate to a call for help in Emacs. But you really want it to behave by deleting the previous character, which is the default for the DEL key in Emacs. Why? Who knows.
It's not quite as important now for me, because I use a terminal program to connect to my remotely hosted box now, and it does the remapping for me.
Anyway, there's a function that plays some serious games with this and does it in a simpler way than mucking about with the translate table. Check out 'normal-erase-is-backspace-mode' - very interesting routine.
Man, Emacs has almost as many nooks and crannies as FreeBSD!
It's not quite as important now for me, because I use a terminal program to connect to my remotely hosted box now, and it does the remapping for me.
Anyway, there's a function that plays some serious games with this and does it in a simpler way than mucking about with the translate table. Check out 'normal-erase-is-backspace-mode' - very interesting routine.
Man, Emacs has almost as many nooks and crannies as FreeBSD!
Monday, July 18, 2005
Cable modem tricks
I recently moved (back) to a cable modem, after being, pretty happily, with Speakeasy DSL. I had used cable modems since they first came out, but moved to DSL because of the much faster upload speeds. As I work for a video conferencing software company (inSORS), upload speeds are as important as download ones. And I could get a 1mb SDSL (synchronous DSL, so upload & download speeds were the same), I made the switch.
However, the cost was pretty steep (US$200 per month), even if my company was paying for it. Now that cable modems are up to 768kpbs upload speeds (and 6mb down!), for about $50 a month, the switch was pretty much a no brainer. I've always told folks that asked that cable is, in general, a much more economically feasible broadband alternative than DSL. For the same speeds, DSL is generally twice as much. Plus you have to deal with two or three different folks (Speakeasy was my provider, Covad did the work and it was actually Verizons "copper" wire in the end).
But I miss Speakeasy. I got some cool perks (free download service, game server time, etc), as well as 4 static IP addresses. For reasons unknown to me and even to folks who know a million times more than me, cable companies have always gone with a dynamic IP address. While it almost never seems to change, they are free to give you a different IP address at any time. So running servers and the like is a little more difficult. You can go with a dynamic dhs host (I used to use TZO), it's still much more of a pain. Speakeasy was very server friendly. Instead, I relocated my servers to my friend's ISP (MV.COM if you're interested), and solved all kinds of problems that way!
Anyway, here's a cool page with lots of cable modem tips. I recently found out that the IP address 192.168.100.1 connects to my cable modem and shows some basic info about it. That's easy and this page shows even more stuff like that.
Cable Modem Troubleshooting Tips: IP addresses
However, the cost was pretty steep (US$200 per month), even if my company was paying for it. Now that cable modems are up to 768kpbs upload speeds (and 6mb down!), for about $50 a month, the switch was pretty much a no brainer. I've always told folks that asked that cable is, in general, a much more economically feasible broadband alternative than DSL. For the same speeds, DSL is generally twice as much. Plus you have to deal with two or three different folks (Speakeasy was my provider, Covad did the work and it was actually Verizons "copper" wire in the end).
But I miss Speakeasy. I got some cool perks (free download service, game server time, etc), as well as 4 static IP addresses. For reasons unknown to me and even to folks who know a million times more than me, cable companies have always gone with a dynamic IP address. While it almost never seems to change, they are free to give you a different IP address at any time. So running servers and the like is a little more difficult. You can go with a dynamic dhs host (I used to use TZO), it's still much more of a pain. Speakeasy was very server friendly. Instead, I relocated my servers to my friend's ISP (MV.COM if you're interested), and solved all kinds of problems that way!
Anyway, here's a cool page with lots of cable modem tips. I recently found out that the IP address 192.168.100.1 connects to my cable modem and shows some basic info about it. That's easy and this page shows even more stuff like that.
Cable Modem Troubleshooting Tips: IP addresses
Monday, July 11, 2005
Practice Healthy Computing
I've been looking for something to keep a more active watch on my server, which is now kept remotely. Here's one possiblity. I need to find out if the motherboard has any of the supported chips, though.
Port description for sysutils/healthd
Port description for sysutils/healthd
Script for setting up internet sharing
Here's a script that will help set up your machine by asking questions about your internet sharing and firewalling. Looks pretty cool:
IPFilter "automagic' script for FreeBSD / Roq.com
IPFilter "automagic' script for FreeBSD / Roq.com
Sunday, July 10, 2005
More on upgrading perl
Here's a page with more info on the perl upgrade script that I mentioned earlier:
Upgrading Perl On FreeBSD - freebsd.munk.nu
Upgrading Perl On FreeBSD - freebsd.munk.nu
Friday, July 8, 2005
Playing A Little Night Music
If you want to play a music cd, you don't need to mount it. But you do need permission to access the device. So, as root, type:
To make this a more permanent change, add this to /etc/devfs.conf:
so it will set the correct permissions at boot.
$ chmod 666 /dev/acdo
To make this a more permanent change, add this to /etc/devfs.conf:
perm acd0 0666
so it will set the correct permissions at boot.
Reinstall boot manager
Say FreeBSD forgets that you have Windows (boo hiss) installed on hard drive, and yet you want to continue to dual boot. Here's some easy steps to either get BootMgr running again, or to start it running for the first time:
1) Do as root "sysctl kern.geom.debugflags=16"
2) Start sysinstall
3) Go Configure->FDISK-> "OK" -> Q
4) It will ask if you wish boot manager
5) Select BootMgr
Thursday, July 7, 2005
Install walkthrough
Nice step by step procedeure for installing FreeBSD, with lots of other links:
How To Install FreeBSD OS Primer
How To Install FreeBSD OS Primer
Don't fsck me
If you want to tell FreeBSD to not run fsck at boot time on a particular partition (maybe you are sharing it with another OS, or maybe it just isn't worth the trouble), you can set the sixth (last) field in /etc/fstab to be 0 (zero). This field is the order for running fsck, and if it is zero, don't do it at all.
fstab man page
fstab man page
Wednesday, July 6, 2005
I can hear your heartbeat
Now that my system is stored remotely, I'm looking into some way of keeping track of its status. One way is a "heartbeat", which regularily pings it and sends me a message if there is a problem. Here's one implementation in ports:
Port description for sysutils/heartbeat
Port description for sysutils/heartbeat
Thursday, June 30, 2005
What are "Load Averages"?
Cool, if very technical, article on 'load averages' and how Unix figures these out. You can see them if you use top, and check out some of the numbers at the top.
UNIX Load Average Part 1: How It Works by Dr. Neil Gunther
UNIX Load Average Part 1: How It Works by Dr. Neil Gunther
Upgrading Perl
If you are going to upgrade perl (currently, if you go to 5.8.7), be sure to read the /usr/ports/UPDATING note:
In other words:
run "perl-after-upgrade" (note the remarks)
run "perl-after-upgrade -f" (to make the changes)
20050624:
AFFECTS: users of lang/perl5.8
lang/perl5.8 has been updated to 5.8.7. You should update
everything depending on perl. The easiest way to do that is
to use perl-after-upgrade script supplied with lang/perl5.8.
Please see its manual page for details.
In other words:
run "perl-after-upgrade" (note the remarks)
run "perl-after-upgrade -f" (to make the changes)
Tuesday, June 28, 2005
Syncing /usr/ports
Say you have two machines that you want to keep up to date with /usr/ports. Rather than running cvsup on both of them, you have a couple of options:
- Use rsync for /usr/ports from the fast machine to the slower machine.
- Use pkg_create's -b option, then use those packages on the other machine(s).
Stop package from upgrading
Question: How do I tell portupgrade to not upgrade a particular port? Say, an upgraded app breaks on my system and I want it to be ignored when I do an "upgrade all" via portupgrade.
Answer:
Answer:
- Use the '-x' option
- Long term solution - HOLD_PKGS in /usr/local/etc/pkgtools.conf
Thursday, June 16, 2005
Keeping FreeBSD up to date
An excellent article on keeping FreeBSD up to date, especially remotely (as I will be doing in the next few days):
TaoSecurity: Keeping FreeBSD Up-To-Date
TaoSecurity: Keeping FreeBSD Up-To-Date
Friday, April 29, 2005
Tuesday, April 5, 2005
HZ Value
There's been some discussion on the freebsd-questions mailing list about the "HZ" value you can set in the kernel. It basically is a setting for how often the kernel gets invoked to do its kernel thing. HZ=100 is a very common and normal setting, it can be modified. Here's a great posting by 'cpghhost' about it:
On Mon, Apr 04, 2005 at 11:17:19AM +0200, Dick Hoogendijk wrote:
>> I read about polling and HZ=1000. The latter is not high enough for
>> vmware3 (it complains..). I read 'man polling' and learn something about
>> this, but I really want to know more about 'hz=1000'
>>
>> The GENERIC has hz=100 as I recall (fbsd-4.11). Can somebody explain I
>> "human language" what happens if you raise this HZ? Is there a processor
>> related issue here? What exectly happens if you have 'hz=1000' or is it
>> maybe better to have 'hz=2000' ? I can't decide now because I don't
>> understand what happens.. So, anybody?
This is the frequency of the heart beat. Superficially, every 1/HZ second,
a clock tick happens and the kernel is invoked. Then stuff like cleanup and
scheduling a new thread happens (this is not the only time when threads
get scheduled though).
HZ=100 is good enough for most purposes. If the kernel needs to poll
some data faster and more frequently, you can crank up HZ to 1000 or
even higher. A system with higher HZ is usually also more responsive,
but this comes at a price:
* The overhead is higher (you need more time to do internal housekeeping,
and there's less time available for userland threads)
* Processor caches are flushed more frequently, resulting in slower
RAM access.
A high value for HZ is also not a good idea for slow(er) hardware
either.
Interestingly, HZ=100 has remained constant for decades (!), despite
CPUs getting faster all the time. This is an excellent value for most
typical usage patterns. Cranking it up should only be required for
special cases. Anyway, the HZ knob is there. Experiment with it until
you get optimal performance.
Tuning your FreeBSD installation
A good place to start when you begin the move to tweaking your FreeBSD system performance is the tuning(7) man page:
tuning
tuning
Friday, April 1, 2005
Yet Another FreeBSD page
Very helpful looking web site, documenting a user's experience with FreeBSD. Much like my site here!
Roland's FreeBSD page
Roland's FreeBSD page
Tuesday, March 29, 2005
Installing 5.3
As I'm going to try and do the install of 5.3, I'm digging up some links to follow. I downloaded the miniinst ISO, just to make it a little easier. We'll see what happens from there.
Here's what I've found so far:
I'll add any more links I come across to this entry.
Here's what I've found so far:
- Of course, you need to start here : Installing FreeBSD, which is the installation guide from the FreeBSD.org handbook.
- Installing FreeBSD 5.3-RELEASE
from the Colorado State engineering department's very useful looking FreeBSD Guide.
FreeBSD Stable Release Install Guide : It is a 4.9/4.10 install guide, but it is very well done.
I'll add any more links I come across to this entry.
FreeBSD Guide page
Here's a very useful looking web site called "FreeBSD Guide". It looks like it is hosted by the Colorado State engineering department. Lots of cool help found here so far!
Welcome to our FreeBSD Guide!
Welcome to our FreeBSD Guide!
Live CD list
The LiveCD List is a table with all the myriad possible "Live" CDs that are downloadable. 99% of them are, of course, Linux ones, but both the FreeBSD Live CD and the FreeSBIE cd are on the list. It's a great way to test out your configuration, and many of them can be installed right from the LiveCD. Check it out!
The LiveCD List
The LiveCD List
Trying 5.3 again
Well, I'm going to try it again. I'm downloading the latest 5.3 released version, and I'm going try to installed 5.3 on my "other" machine. As mentioned in here previously, I haven't had much luck with a full-blown, X Windows install, having problems with both X and getting the mouse to work through my KVM switch. But the fact that the FreesBie release worked pretty well has encouraged me to try it again. We'll see, I guess.
Sunday, March 27, 2005
Wiki overview
I'm looking into adding a Wiki to some of the web sites I host, and I came across this pretty solid overview of open source wiki software. Written in Nov. 2004, it's pretty up to date and has given me lots of grist to chew over:
ONLamp.com: Which Open Source Wiki Works For You?
ONLamp.com: Which Open Source Wiki Works For You?
Saturday, March 12, 2005
More IPFiltter help
Yet another page, somewhat dated, for using IPFilter:
Firewalling with FreeBSD and IPF
Of course, don't forget the handbook page:
Firewalls
And your local examples found at:
/usr/share/examples/ipfilter/
Firewalling with FreeBSD and IPF
Of course, don't forget the handbook page:
Firewalls
And your local examples found at:
/usr/share/examples/ipfilter/
Friday, February 18, 2005
The Fish - an rc.conf editor
A program to more visually edit the rc.conf file. While editing it is pretty easy (I'm an Emacs guy, not a vi guy), this program also tells you about all the options you don't know about too!
The Fish for FreeBSD
The Fish for FreeBSD
Cool Hacks post
A cool little column by the author of the way cool little book, BSD Hacks. This one is a short collection of new little notes and hacks for FreeBSD:
ONLamp.com: FreeBSD Tips and Tricks for 2005
ONLamp.com: FreeBSD Tips and Tricks for 2005
Subscribe to:
Posts (Atom)